Hello world program in Java
Hello world program in Java
Program
public class HelloWorld
{
public static void main(String args[])
{
System.out.println("Hello World!");
}
};
Output
Hello World!
Explanation
Let's see what is the meaning of class, public, static, void, main, String[], System.out.println().
- class keyword is used to declare a class in java.
- public keyword is an access modifier which represents visibility, it means it is visible to all.
- static is a keyword, if we declare any method as static, it is known as static method. The core advantage of static method is that there is no need to create object to invoke the static method. The main method is executed by the JVM, so it doesn't require to create object to invoke the main method. So it saves memory.
- void is the return type of the method, it means it doesn't return any value.
- main represents startup of the program.
- String[] args is used for command line argument. We will learn it later.
- System.out.println() is used print statement.
What is System.out.println();
System.out.println is a Java statement that prints the argument passed, into the System.out which is generally stdout.System – is a final class in java.lang package. As per javadoc, “…Among the facilities provided by the System class are standard input, standard output, and error output streams; access to externally defined properties and environment variables; a means of loading files and libraries; and a utility method for quickly copying a portion of an array…“
out – is a static member field of System class and is of type PrintStream. Its access specifiers are public final. This gets instantiated during startup and gets mapped with standard output console of the host. This stream is open by itself immediately after its instantiation and ready to accept data.
println – is a method of PrintStream class . println prints the argument passed to the standard console and a newline. There are multiple println methods with different arguments (overloading). Every println makes a call to print method and adds a newline. print calls write() and the story goes on like that.
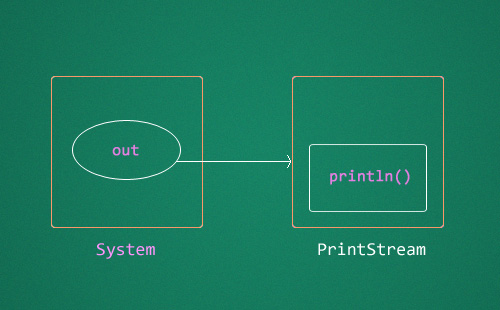
if you are bit lazy about writting System.out.println everytime you can import System.out from java.lang library.
Hello world program with static import
Program
import static java.lang.System.out;
public class HelloWorld
{
public static void main(String args[])
{
out.println("Hello World!");
}
};
Output
Hello World!
Comments
Post a Comment